17. Definite Integrals, Part 1: The Building Blocks¶
Expanded on Monday, March 8, adding an Appendix on the Left-hand Endpoint Rule
References:
Sections 5.2.1, 5.2.3 and 5.2.4 of Sauer
Sections 4.3 and 4.4 of Burden&Faires
17.1. Introduction¶
The objective of this and several subsequent sections is to develop methods for approxmating a definite integral
This is arguably even more important than approximating derivatives, for several reasons; in particualr, because there are many functions for which antiderivative formulas cannot be found, so that the result of the Fundamental Theorem of Calculus, that
does not help us.
One core idea is to approximate the function \(f\) by a polynomial (or several), and use its integral as an approximation. The two simplest possibilities here are approximating by a constant and by a straight line; here we explore the latter; th former wil be visited soon.
from numpy import array, linspace, exp
from matplotlib.pyplot import figure, plot, title, grid, legend
17.2. Approximating with a single linear function: the Trapezoid Rule¶
The idea is to approximate \(f:[a, b] \to \Bbb{R}\) by collocation at the end points of this interval:
Then the approximation — which will be called \(T_1\), for reasons that will becom clear soon — is
This can be interpreted as replacing \(f(x)\) by \(f_{ave}\) the average of the value at the end points, and inegrting that simple function.
For the example \(f(x) = e^x\) on \([-1, 3]\)
a = 1
b = 3
def f(x): return exp(x)
f_ave = (f(a) + f(b))/2
x = linspace(a, b)
figure(figsize=[12, 8])
plot(x, f(x))
plot([a, a, b, b, a], [0, f(a), f(b), 0, 0], label="Trapezoid Rule")
plot([a, a, b, b, a], [0, f_ave, f_ave, 0, 0], '-.', label="Trapezoid Rule area")
legend()
grid(True)
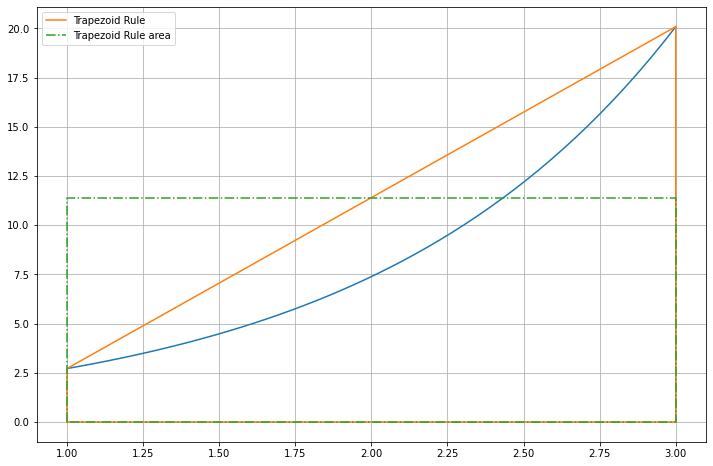
The approximation \(T_1\) is the area of the orange trapezoid (hence the name!) which is also the area of the green rectangle.
17.3. Approximating with a constant: the Midpoint Rule¶
The idea here is to approximate \(f:[a, b] \to \Bbb{R}\) by its value at the midpoint of the interval, like the building blocks in a Riemann sum with the middel being the intuitive best choice of where to put the rectangle.
Then the approximation — which will be called \(M_1\) — is
For the same example \(f(x) = e^x\) on \([-1, 3]\)
f_mid = f((a+b)/2)
figure(figsize=[12, 8])
plot(x, f(x))
plot([a, a, b, b, a], [0, f_mid, f_mid, 0, 0], 'r', label="Midpoint Rule")
grid(True)
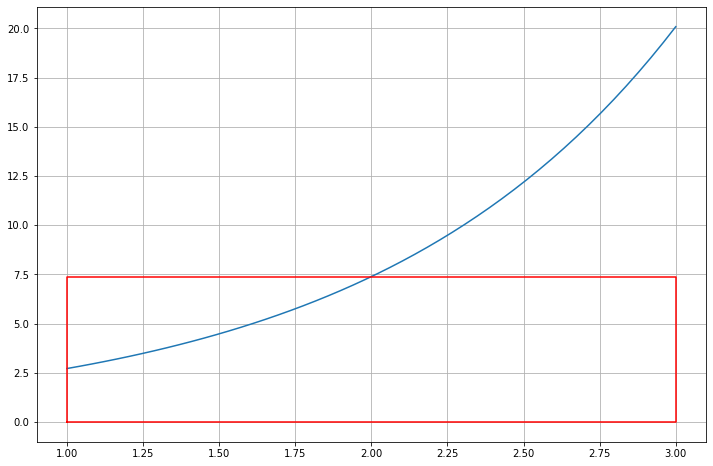
The approximation \(M_1\) is the area of the red rectangle.
The two methods can be compared my combining these graphs:
f_mid = f((a+b)/2)
figure(figsize=[12, 8])
plot(x, f(x))
plot([a, a, b, b, a], [0, f(a), f(b), 0, 0], label="Trapezoid Rule")
plot([a, a, b, b, a], [0, f_ave, f_ave, 0, 0], '-.', label="Trapezoid Rule area")
plot([a, a, b, b, a], [0, f_mid, f_mid, 0, 0], 'r', label="Midpoint Rule")
legend()
grid(True)
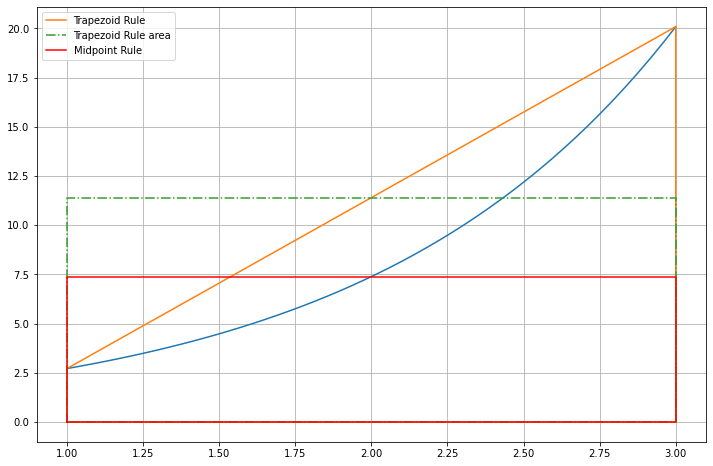
17.4. Error Formulas¶
These graphs indicate that the trapezoid rule will over-estimate the error for this and any function that is convex up on the interval \([a, b]\). With closer examination it can perhaps be seen that the Midpoint Rule will instead underestimate in this situation, because its “overshoot” at left is less than its “undershoot” at right.
We can derive error formulas that confirm this, and which are the basis for both practical error estimates and for deriving more accurate approximation methods.
The first such method will be to use multiple small intervals instead of a single bigger one (using piecewise polynomial approximation) and for that, it is convenient to define \(h = b-a\) which will become the parameter that we reduce in order to improve accuracy.
Theorem 1. Error in the Trapezoid Rule, \(T_1\)
For a function \(f\) that is twice differentiable on interval \([a, b]\), the error in the Trapezoid Rule is
It will be convenient to define \(h := b-a\) so that this becomes
Theorem 2. Error in the Midpoint Rule, \(M_1\)
For a function \(f\) that is twice differentiable on interval \([a, b]\) and again with \(h=b-a\), the error in the Midpoint Rule is
These will be verified below, using the error formulas for Taylor polynomials and collocation polynomials.
For now, note that:
The results confirm that for a function that is convex up, the Trapezoid Rule overestimates and the Midpoint Rule underestimates.
The ratio of the errors is approximately \(-2\). This will be used to get a better result by using a weighted average: Simpson’s Rule.
The errors are \(O(h^3)\). This opens the door to Richardson Extrapolation, as will be seen soon in the method of Romberg Integration.
17.4.1. Proofs of these error results¶
One side benefit of the following verifications is that they also offer illustrations of how the two fundamental error formulas help us: Taylor’s Formula and its cousin the error formula for polynomial collocation.
17.4.1.1. Proof of Theorem 1: the error in the Trapezoid Rule¶
The function integrated to get the Trapezoid Rule is the linear collocating polynomial \(L(x)\), and from the section Error Formulas for Polynomial Collocation we have
Integrating each side gives
Remember that \(\xi_x\) depends on \(x\) in an unknown way; to get around that complication, we introduce a result that also helps in various places later:
Theorem 3. The Integral Mean Value Theorem
In an integral
with \(f\) continuous and the “weight function” \(w(x)\) positive valued (actually, it is enough that \(w(x) \geq 0\) and it is not zero everyhere), there is a point \(\xi \in [a,b]\) that gives a “weighted average value” for \(f(x)\) in the sense that
Proof:
As \(f\) is continuous on the closed, bounded interval \([a, b]\), the Extreme Value Theorem from calculus says that \(f\) has a minimum \(L\) and a maximum \(H\) on this interval: \(L \leq f(x) \leq H\). Since \(w(x) \geq 0\), this gives
and by integrating,
Dividing by \(\int_a^b w(x) \,dx\) (which is positive),
and the Mean Value Theorem says that \(f\) attains this value for some \(\xi \in [L, H]\):
Clearing the denominator gives the claimed result.
Returning to Theorem 1, we use this with weight function \(w(x) = (x-a)(b-x), \geq 0\) for \(a \leq x \leq b\). Then with \(-f''\) as the function \(f\) in the above formula,
A bit of calculus gives \(\displaystyle \int_a^b (x-a)(b-x) \, dx = \frac{(b-a)^3}{6}\), so
as advertised.
17.4.1.2. Proof of Theorem 2: the error in the Midpoint Rule¶
For this, we can use Taylor’s Theorem for the linear approximation
with \(c = (a+b)/2\), the midpoint. That is,
and integrating each side gives
Here symmetry helps, by eliminating the first (potentialy biggest) term in the error: we use the fact that \(a = c - h/2\) and \(b = c + h/2\)
Thus the error simplifies to
and much as above, the Integral Mean Value Theorem can be used, this time with weight function \(w(x) = (x-c)^2, \geq 0\):
Another caluclus exercise: \(\displaystyle \int_a^b (x-c)^2 dx = \int_{-h/2}^{h/2} x^2 dx = \left[x^3/3\right]_{-h/2}^{h/2} = h^3/12\), so indeed,
17.5. Appendix: Approximating a Definite Integral With the Left-hand Endpoint Rule¶
An even simpler approximation of \(\int_a^b f(x)\, dx\) is the Left-hand Endpoint Rule, probably seen in a calculus course. For a single interval, this uses the approximation
leading to
The correpsonding composite rule with \(n\) sub-intervals of equal width \(h = (b-a)/n\) is
with \(x_i = a + i h\) as before.
Theorem 3. Error in the Left-hand Endpoint Rule, \(L_1\)
For a function \(f\) that is differentiable on interval \([a, b]\), the error in the Left-hand Endpoint Rule is
17.5.1. Proof:¶
This time use Taylor’s Theorem just for the constant approximation wth center \(a\):
That is,
so integrating each side gives
Using the Integral Mean Value Theorem again, now with weight \(w(x) = x-a\) gives
and inserting this into the previous formula gives the result.
This work is licensed under Creative Commons Attribution-ShareAlike 4.0 International